
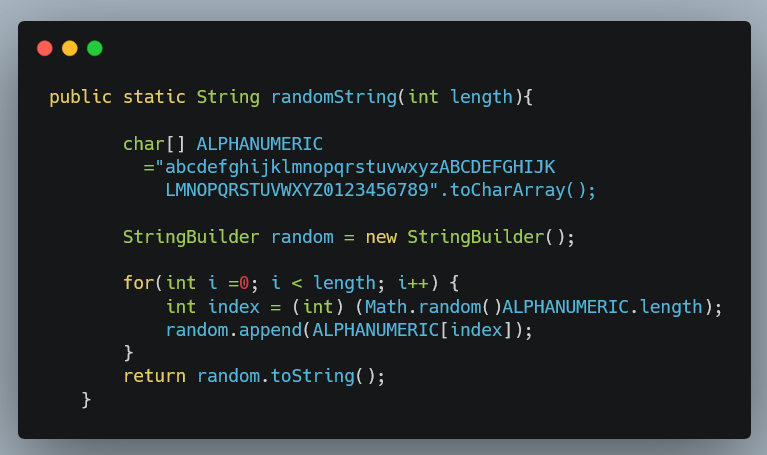
Int answer7 = (int) (Math.random() * n) + min Random random new Random () int array random.ints (100000, 10,100000).toArray () you can print the array and you'll get 100000 random integers. something like this will work, depends if you want double or ints etc. Int answer6 = (int) (Math.random() * n) + min You can use IntStream ints () or DoubleStream doubles () available as of java 8 in Random class. Int answer5 = (int) (Math.random() * n) + min Int answer4 = (int) (Math.random() * n) + min Int answer3 = (int) (Math.random() * n) + min Int answer2 = (int) (Math.random() * n) + min When this method is first called, it creates a single new pseudorandom-number generator, exactly as if by the expression. Returned values are chosen pseudorandomly with (approximately) uniform distribution from that range. Int answer1 = (int) (Math.random() * n) + min The () returns a double value with a positive sign, greater than or equal to 0.0 and less than 1.0. Int answer = (int) (Math.random() * n) + min This Will work when exciuted, and its done in a simple manner such as what you provided import ("Printing 10 random integers from 1 to n.") * Lesson 8 Coding Activity Question 1 */
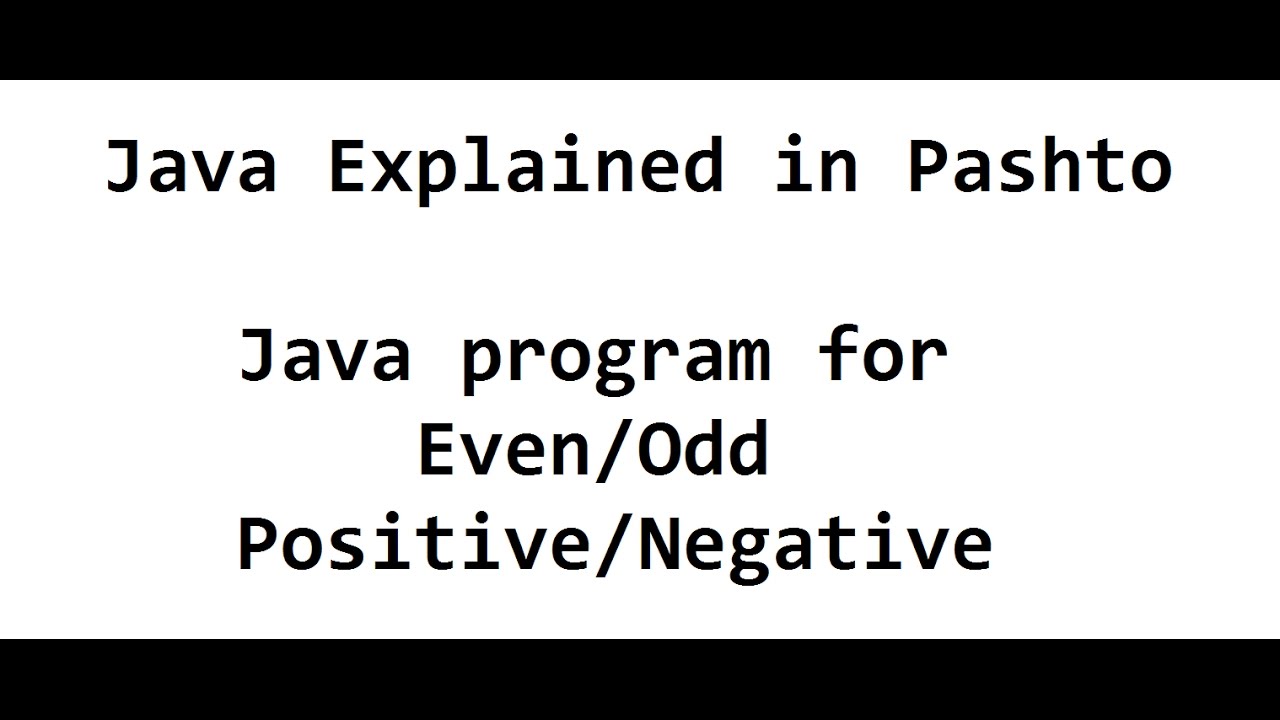
How do I make it so that the output is different every time? As of now every output following the first one is exactly the same.
#Math.random java negative or positive code#
You want to do this by shifting (actually division and multiplication, but because we're dealing with a power of 2 we can do it with bit shifts) and not with rounding (which is what masking is essentially).Īs a general rule of thumb, you don't want to throw away random bits when you don't have to.Write the code to ask the user for a positive integer n, then print 10 random integers from 1 to n inclusive using Math.random().
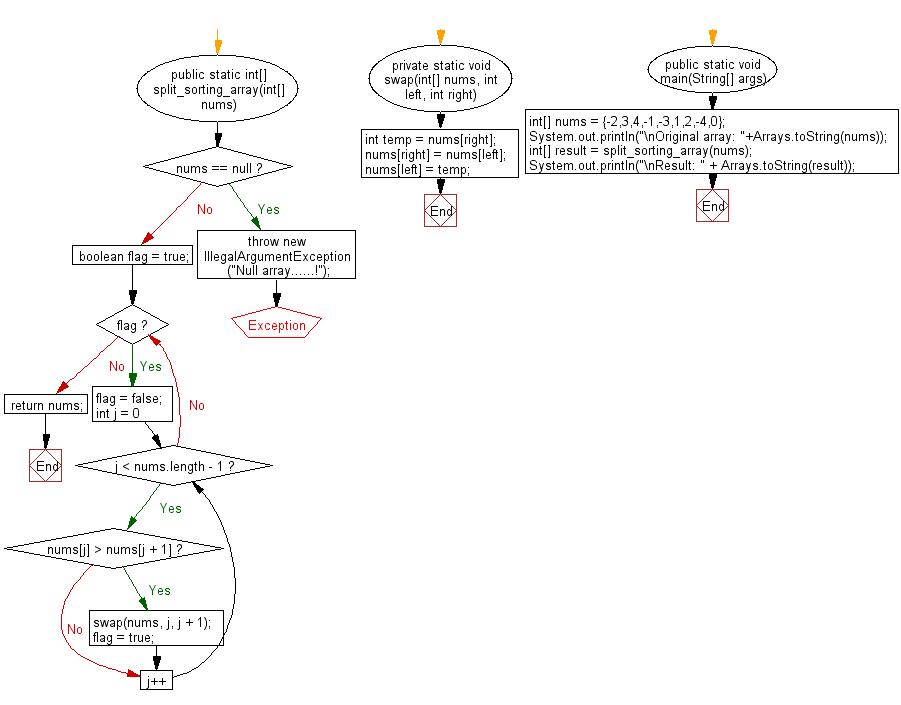
Instead, you want to provide a min and max and find an even number in that range. This can throw you for a loop (no pun intended) by causing them to be slightly biased. random.nextInt () << 1 Shift left by one bit using multiplication. There are an odd number of positive and an odd number of negative values. To generate even numbers, just do any of the following: Mask off the least significant bit ( -2 is 0xFFFFFFFE, or a bitmask with all ones except for the last bit) random.nextInt () & -2 Shift left by one bit using the bit-shift operator. You do not want to mask to make your elements even. You should therefore use a private static variable to store the Random object.
#Math.random java negative or positive generator#
The pseudorandom number generator actually carries some state, even if you don't think of it that way. It's bad practice to create a new instance of Random every time you want to generate one random number, though. In that case, the whole question about helper functions would be irrelevant. That would be more efficient than looping, testing, and discarding. To generate a random even number, you could just take random.nextInt() & -2 to mask off the least significant digit. There is a convention in Java that functions named isSomething() return a boolean and have no side effects. I would also rename evenNumber(int number) to isEven(int number). Therefore, the compiler has enough of a hint that it could decide to inline the entire function. The combination of those three keywords means that the code would be unaffected by any instance variable, any superclass, or any subclass, and is also not callable by any code external to the class. I would go one step further and declare your functions as private static final, if possible.
